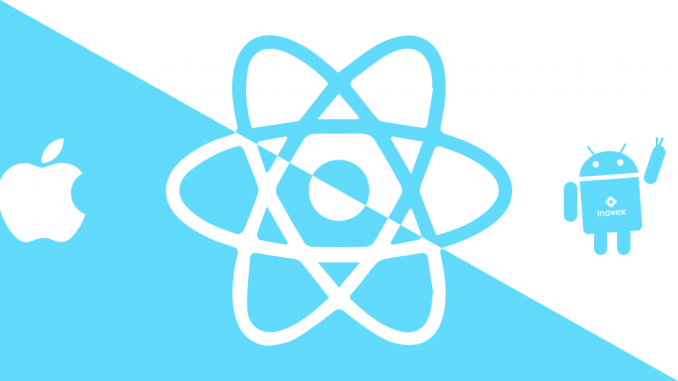
import React, { useState } from 'react';
import { View, TextInput, Button } from 'react-native';
import axios from 'axios';
const YourScreen = () => {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const sendData = async () => {
try {
const response = await axios.post('http://your-php-server/save_data.php', {
name: name,
email: email,
});
console.log(response.data);
} catch (error) {
console.error(error);
}
};
return (
<View>
<TextInput
placeholder="Name"
value={name}
onChangeText={(text) => setName(text)}
/>
<TextInput
placeholder="Email"
value={email}
onChangeText={(text) => setEmail(text)}
/>
<Button title="KIRIM" onPress={sendData} />
</View>
);
};
export default YourScreen;
Sementara itu script PHP-nya akan nampak seperti berikut :
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database_name";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check the connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Get the data from the request
$name = $_POST['name'];
$email = $_POST['email'];
// Perform any necessary validation or sanitization on the data
// Prepare the SQL statement
$sql = "INSERT INTO your_table_name (name, email) VALUES (?, ?)";
$stmt = $conn->prepare($sql);
// Bind parameters and execute the statement
$stmt->bind_param("ss", $name, $email);
$stmt->execute();
// Check if the query was successful
if ($stmt->affected_rows > 0) {
echo "Data saved successfully";
} else {
echo "Failed to save data";
}
// Close the statement and connection
$stmt->close();
$conn->close();
?>
Leave a Reply