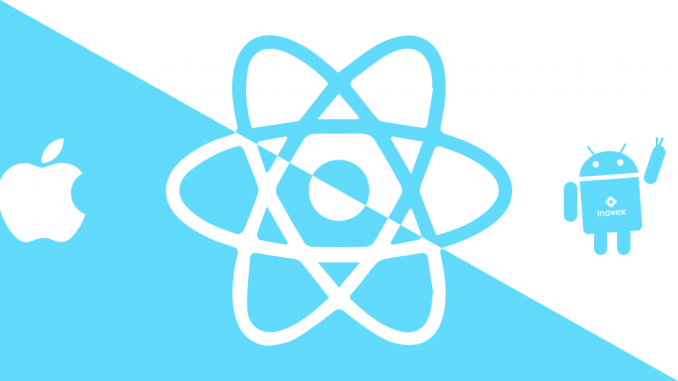
Pertama kita buat script PHP untuk Fetch Data :
<?php
// Database credentials
$dbHost = 'localhost';
$dbName = 'your_database_name';
$dbUser = 'your_username';
$dbPass = 'your_password';
// Create a connection
$conn = mysqli_connect($dbHost, $dbUser, $dbPass, $dbName);
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Fetch data from the database
$sql = "SELECT * FROM your_table_name";
$result = mysqli_query($conn, $sql);
$data = array();
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
$data[] = $row;
}
}
// Close the connection
mysqli_close($conn);
// Return the data as JSON
header('Content-Type: application/json');
echo json_encode($data);
?>
2. Lakukan permintaan jaringan dari React Native: Pada aplikasi React Native Anda, lakukan permintaan jaringan ke skrip PHP menggunakan API fetch atau librari seperti Axios. Permintaan ini akan mengambil data dari skrip PHP.
import React, { useEffect, useState } from 'react';
import { View, Text } from 'react-native';
const MyComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetchData();
}, []);
const fetchData = () => {
fetch('http://your-php-server-url/your-php-script.php')
.then(response => response.json())
.then(data => {
setData(data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
};
return (
<View>
{data.map(item => (
<Text key={item.id}>{item.name}</Text>
))}
</View>
);
};
export default MyComponent;
Leave a Reply